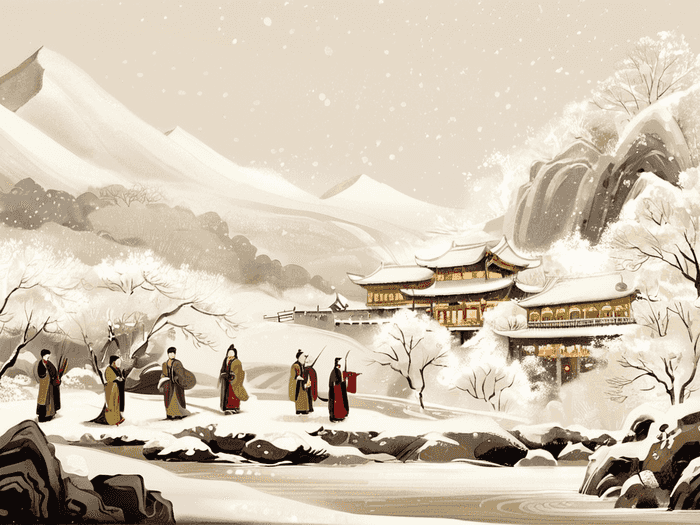
面试考官邢老师为您分享以下优质知识
在C语言中读入二进制文件内容的基本步骤如下:
一、打开文件
使用`fopen`函数以二进制读取模式(模式为`"rb"`)打开文件。若文件打开失败,`fopen`将返回`NULL`指针,需进行错误检查。
```c
FILE *fp = fopen("filename.bin", "rb");
if (fp == NULL) {
perror("Failed to open file");
return 1;
}
```
二、读取文件内容
使用`fread`函数将数据从文件读取到内存中。需指定存储数据的指针、每个数据项的大小、要读取的项数以及文件指针。
示例:读取基本数据类型
```c
int array;
size_t num_elements = fread(array, sizeof(int), 100, fp);
if (num_elements == 0) {
perror("Failed to read file");
fclose(fp);
return 1;
}
```
示例:读取结构体数据
```c
struct mystruct {
float value;
char name;
};
struct mystruct buffer;
size_t count = fread(buffer, sizeof(struct mystruct), 10, fp);
if (count == 0) {
perror("Failed to read file");
fclose(fp);
return 1;
}
```
三、关闭文件
使用`fclose`函数关闭文件,释放系统资源。
```c
fclose(fp);
```
四、完整示例
以下是一个完整的示例,展示如何读取二进制文件中的整数数组并打印:
```c
include
include
int main() {
FILE *fp;
int array;
size_t num_elements;
// 打开文件
fp = fopen("data.bin", "rb");
if (fp == NULL) {
perror("Failed to open file");
return 1;
}
// 读取数据
num_elements = fread(array, sizeof(int), 100, fp);
if (num_elements == 0) {
perror("Failed to read file");
fclose(fp);
return 1;
}
// 打印数据
for (size_t i = 0; i < num_elements; i++) {
printf("%dn", array[i]);
}
// 关闭文件
fclose(fp);
return 0;
}
```
注意事项
读取时需确保内存缓冲区大小与文件中数据类型匹配,否则可能导致数据损坏。
建议对`fopen`、`fread`的返回值进行检查,避免程序崩溃。
使用`%s`格式化输出二进制数据时需注意字符编码问题,建议使用`%d`等类型化输出。
通过以上步骤,可安全高效地读入二进制文件内容。