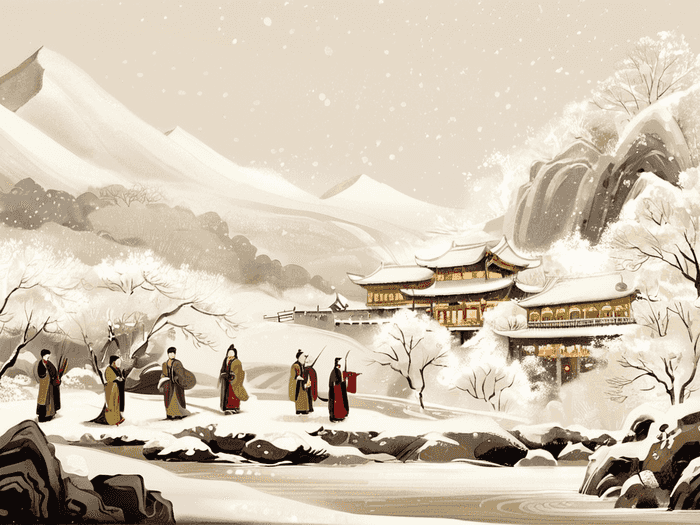
随梦而飞为您分享以下优质知识
根据搜索结果,您提到的“二进制码树”可能指二叉树(Binary Tree)。以下是创建和打印二叉树的常见方法,以Java为例:
一、二叉树节点定义
首先,定义二叉树的节点结构,包含数据域和左右子节点指针:
```java
class TreeNode {
int data;
TreeNode left, right;
TreeNode(int data) {
this.data = data;
left = right = null;
}
}
```
二、插入节点方法
实现插入节点的功能,根据方向(左或右)将新节点添加到树中:
```java
void add(TreeNode root, int value, char direction) {
if (root == null) {
root = new TreeNode(value);
return;
}
if (direction == 'L' && root.left == null) {
root.left = new TreeNode(value);
} else if (direction == 'R' && root.right == null) {
root.right = new TreeNode(value);
} else {
throw new IllegalArgumentException("Invalid direction or node already exists.");
}
}
```
三、中序遍历打印树
通过中序遍历(左-根-右)打印二叉树,可验证树结构:
```java
void inorderTraversal(TreeNode root) {
if (root != null) {
inorderTraversal(root.left);
System.out.print(root.data + " ");
inorderTraversal(root.right);
}
}
```
四、完整示例
以下是整合上述功能的完整代码示例:
```java
import java.util.Scanner;
public class BinaryTreeExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
TreeNode root = null;
int n = scanner.nextInt(); // 节点数量
for (int i = 0; i < n; i++) {
int value = scanner.nextInt();
char direction = scanner.next().charAt(0);
add(root, value, direction);
}
System.out.println("中序遍历结果:");
inorderTraversal(root);
scanner.close();
}
static void add(TreeNode root, int value, char direction) {
if (root == null) {
root = new TreeNode(value);
return;
}
if (direction == 'L' && root.left == null) {
root.left = new TreeNode(value);
} else if (direction == 'R' && root.right == null) {
root.right = new TreeNode(value);
} else {
throw new IllegalArgumentException("Invalid direction or node already exists.");
}
}
static void inorderTraversal(TreeNode root) {
if (root != null) {
inorderTraversal(root.left);
System.out.print(root.data + " ");
inorderTraversal(root.right);
}
}
}
```
五、注意事项
确保方向为`L`或`R`,且节点唯一性(避免重复插入)。
中序遍历适用于二叉搜索树,若需其他遍历方式(前序/后序),可调整`inorderTraversal`方法。
若需绘制二叉树的图形化表示,可结合第三方库(如Graphviz)或自定义绘图逻辑实现,但上述代码仅支持结构化存储和遍历。