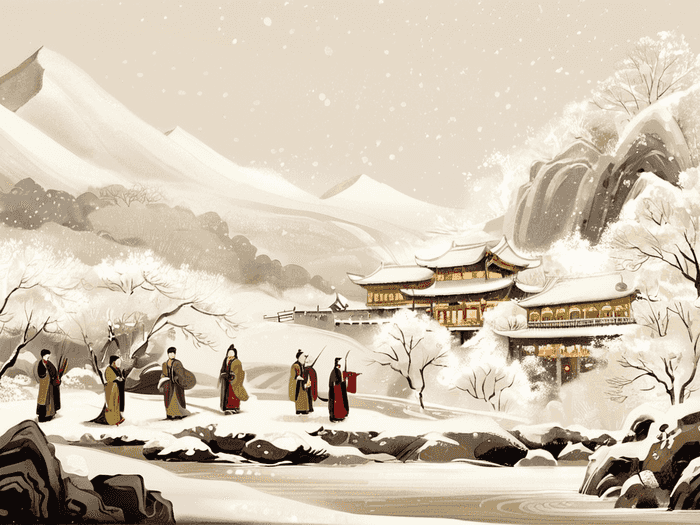
心随风动为您分享以下优质知识
图像转换为二进制数据可以通过多种方式实现,以下是常见的方法及示例代码:
一、使用Python进行图像二进制转换
将图像保存为二进制文件
使用PIL库将图像保存为二进制文件(如PNG或JPEG格式)。
```python
from PIL import Image
def image_to_binary(file_path, output_path):
with Image.open(file_path).convert('RGB') as img:
img.save(output_path, 'PNG') 或 'JPEG'
return open(output_path, 'rb').read()
示例
binary_data = image_to_binary('a.png', 'output.png')
with open('output.png', 'wb') as f:
f.write(binary_data)
```
将图像像素转换为二进制字符串
遍历图像像素,将非白色像素设为'1',白色设为'0',保存为二进制文件。
```python
from PIL import Image
def image_to_binary_pixels(file_path, output_path, size=(32, 32)):
img = Image.open(file_path).convert('RGB')
with open(output_path, 'wb') as f:
for i in range(size):
for j in range(size):
pixel = img.getpixel((j, i))
if pixel != (255, 255, 255):
f.write(b'1')
else:
f.write(b'0')
示例
image_to_binary_pixels('a.png', 'binary_output.txt')
```
二、使用Java进行图像二进制转换
将图像保存为字节数组
使用`ImageIO`读取图像并转换为字节数组。
```java
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.File;
public class ImageToBinary {
public static byte[] getImageData(String imagePath) throws IOException {
BufferedImage image = ImageIO.read(new File(imagePath));
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ImageIO.write(image, "PNG", baos);
return baos.toByteArray();
}
public static void saveImage(byte[] data, String outputPath) throws IOException {
ByteArrayInputStream bais = new ByteArrayInputStream(data);
ImageIO.write(new BufferedImage(32, 32, BufferedImage.TYPE_INT_RGB), "PNG", new File(outputPath));
}
public static void main(String[] args) {
byte[] binaryData = getImageData("d://1.jpg");
saveImage(binaryData, "output.png");
}
}
```
使用base64编码转换
将字节数组转换为base64字符串,便于存储或传输。
```java
import java.awt.image.BufferedImage;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.util.base64;
public class ImageToBinarybase64 {
public static String encodeImage(String imagePath) throws IOException {
BufferedImage image = ImageIO.read(new File(imagePath));
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ImageIO.write(image, "PNG", baos);
return base64.getEncoder().encodeToString(baos.toByteArray());
}
public static void main(String[] args) {
String base64String = encodeImage("test.jpg");
System.out.println(base64String);
}
}
```
三、其他方法
数据库存储
将图像以二进制形式存储到数据库(如BMP或PNG格式)。
图像处理软件
使用Photoshop、GIMP等工具将图像另存为二进制格式。
总结
图像转换为二进制数据的方法多样,可根据需求选择合适的方式。若需进一步处理(如压缩、传输或存储),建议结合具体场景选择最优方案。