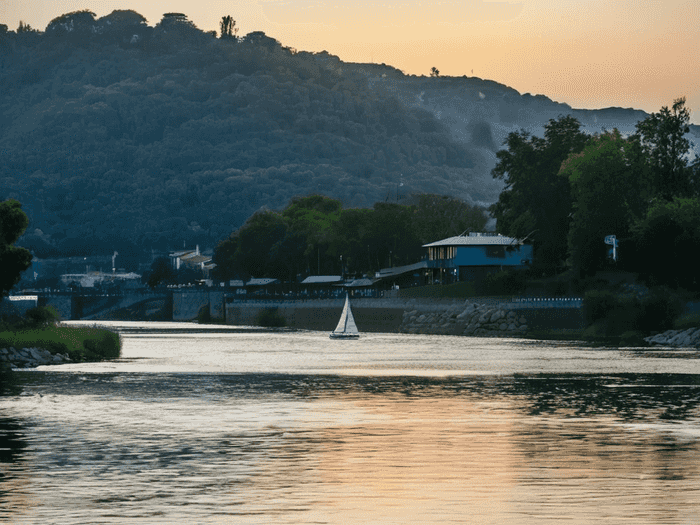
面试考官邢老师为您分享以下优质知识
使用堆栈实现十进制转二进制的方法主要基于 除2取余法,利用堆栈的后进先出(LIFO)特性来存储余数,最终将余数逆序组合成二进制数。以下是具体步骤和示例:
一、基本原理
将十进制数不断除以2,记录每次的余数(0或1),直到商为0。这些余数从下到上排列即为二进制表示。
使用堆栈存储余数,利用`push`操作将余数压入栈中,最后通过`pop`操作依次取出余数并组合成二进制字符串。
二、实现步骤
分配存储空间并设置栈顶指针(通常初始化为-1)。
将十进制数不断除以2,将余数压入堆栈。
依次弹出栈顶元素,将余数拼接成二进制字符串。
三、代码示例
1. C语言实现(顺序栈)
```c
include
include
define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int top;
} SqStack;
void InitStack(SqStack *S) {
S->
top = -1;
}
int Push(SqStack *S, int e) {
if (S->
top == MAXSIZE - 1) {
printf("栈满!n");
return 0;
}
S->
data[++S->
top] = e;
return 1;
}
int Pop(SqStack *S, int *e) {
if (S->
top == -1) {
printf("栈空!n");
return 0;
}
*e = S->
data[S->
top--];
return 1;
}
void Binary(SqStack S) {
int a;
printf("请输入十进制数: ");
scanf("%d", &a);
while (a >
1) {
Push(&S, a % 2);
a = a / 2;
}
Push(&S, 1); // 最高位补1
printf("二进制为: ");
while (!IsEmpty(&S)) {
printf("%d", Pop(&S, &a));
}
printf("n");
}
int main() {
SqStack S;
InitStack(&S);
Binary(S);
return 0;
}
```
2. Java实现(使用`Stack`类)
```java
import java.util.Scanner;
import java.util.Stack;
public class DecimalToBinary {
public static int zhuanhuan(int x) {
Stack stack = new Stack();
while (x != 0) {
stack.push(x % 2);
x = x / 2;
}
int res = 0;
while (!stack.isEmpty()) {
res = res * 10 + stack.pop();
}
return res;
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
System.out.print("请输入一个整数: ");
int x = in.nextInt();
System.out.println("其二进制为: " + zhuanhuan(x));
}
}
```
四、注意事项
需检查栈是否已满,避免溢出。
十进制数最高位为1时,需在结果前补1。
上述方法仅适用于非负整数,负数需单独处理(如补码表示)。
通过上述方法,可高效利用堆栈实现十进制到二进制的转换。