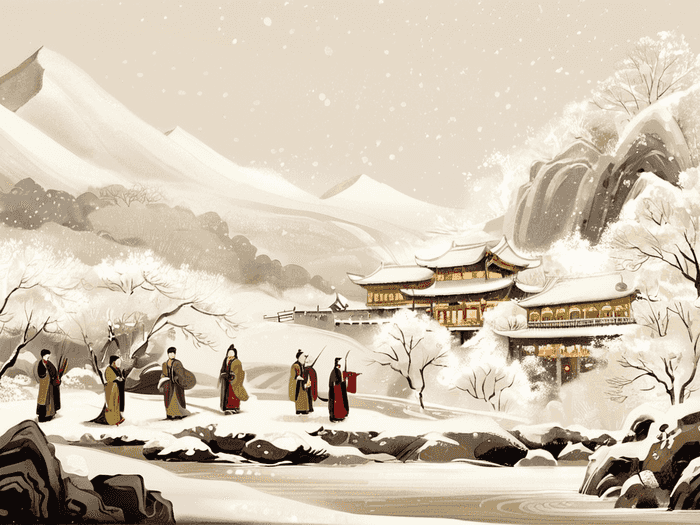
梦幻之旅为您分享以下优质知识
在C语言中,二进制文件读取主要通过以下步骤实现:
一、基本函数与模式
使用 `fopen()` 函数以二进制读取模式打开文件,模式为 `"rb"`(read binary)。
```c
FILE *file = fopen("filename.bin", "rb");
if (file == NULL) {
perror("Failed to open file");
return 1;
}
```
数据读取
使用 `fread()` 函数从文件中读取数据。其原型为:
```c
size_t fread(void *ptr, size_t size, size_t count, FILE *stream);
```
- `ptr`:指向存储数据的缓冲区;
- `size`:每个数据项的大小(如 `sizeof(int)`);
- `count`:要读取的项数;
- `stream`:文件指针。
```c
char buffer;
size_t bytesRead = fread(buffer, sizeof(char), 1024, file);
if (bytesRead == 0) {
perror("Failed to read file");
fclose(file);
return 1;
}
```
关闭文件
使用 `fclose()` 函数关闭文件以释放资源。
```c
fclose(file);
```
二、注意事项
错误处理
- 检查 `fopen()` 是否返回 `NULL`,判断文件是否成功打开;
- 检查 `fread()` 的返回值是否等于预期读取的项数,避免读取不完整。
数据对齐与格式
- 二进制文件按字节顺序存储,需注意数据类型对齐问题。例如,结构体应使用 `pragma pack` 或 `struct` 说明符明确对齐方式。
示例综合
下面是一个完整的示例,读取二进制文件中的整数数组并打印:
```c
include
include
int main() {
FILE *file = fopen("integers.bin", "rb");
if (file == NULL) {
perror("Failed to open file");
return 1;
}
int array;
size_t bytesRead = fread(array, sizeof(int), 100, file);
if (bytesRead == 0 || bytesRead != 100) {
perror("Failed to read file");
fclose(file);
return 1;
}
fclose(file);
printf("Array contents:n");
for (int i = 0; i < 100; i++) {
printf("%d ", array[i]);
}
printf("n");
return 0;
}
```
三、扩展应用
动态读取:
根据实际文件大小动态分配内存,避免固定大小数组导致溢出;
结构体读写:按结构体大小分块读取,例如:
```c
struct MyStruct {
float field1;
int field2;
};
MyStruct *readStruct(FILE *file) {
MyStruct *structPtr = malloc(sizeof(MyStruct));
if (!structPtr) return NULL;
fread(structPtr, sizeof(MyStruct), 1, file);
return structPtr;
}
```
文件头解析:在读取数据前先读取文件头信息(如结构体大小、数据类型等),以便正确解析后续数据。
通过以上方法,可灵活实现二进制文件的读取操作。