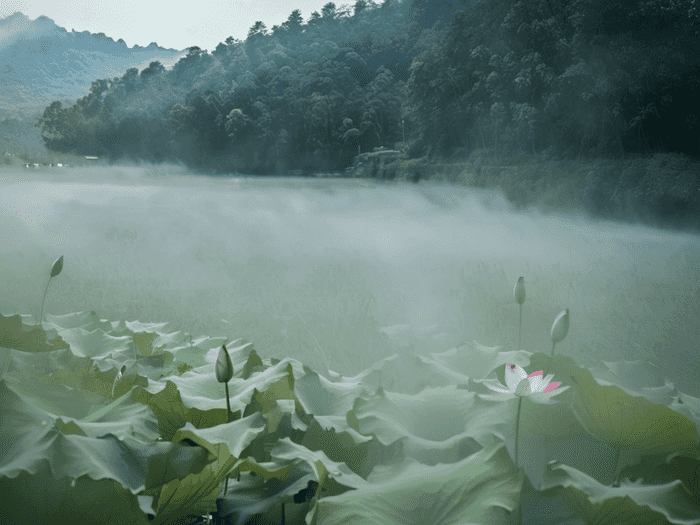
风吹过的记忆为您分享以下优质知识
在C语言中,将二进制数据转换为文件可以通过以下步骤实现,结合了二进制读写和数据格式化输出的方法:
一、二进制数据写入文件
使用 `fwrite()` 函数将结构体或数组以二进制形式写入文件。例如:
```c
include
struct Record {
int x;
float y;
char z;
};
int main() {
Record records = {
{10, 220.5f, "example"},
{20, 330.75f, "data"},
// 添加更多记录...
};
FILE *fp = fopen("data.bin", "wb");
if (fp == NULL) {
perror("Error opening file");
return 1;
}
for (int i = 0; i < 5; ++i) {
fwrite(&records[i], sizeof(Record), 1, fp);
}
fclose(fp);
return 0;
}
```
二、二进制文件转换为文本文件
使用 `fread()` 函数读取二进制数据到数组中。
将二进制数据转换为可读的文本格式(如十六进制或ASCII)并写入新文件。
示例:将二进制数据转换为十六进制文本文件
```c
include
include
void binary_to_hex(const unsigned char *data, int length, char *output) {
const char *hex_table = "0123456789ABCDEF";
for (int i = 0; i < length; ++i) {
sprintf(output + (i * 2), "%02X ", data[i] & 0xFF);
}
output[length * 2] = '0'; // 添加字符串结束符
}
int main() {
FILE *inFile = fopen("data.bin", "rb");
FILE *outFile = fopen("data_hex.txt", "w");
if (inFile == NULL || outFile == NULL) {
perror("Error opening file");
return 1;
}
unsigned char buffer;
size_t bytesRead;
while ((bytesRead = fread(buffer, sizeof(unsigned char), sizeof(buffer), inFile)) >
0) {
binary_to_hex(buffer, bytesRead, outFile);
}
fclose(inFile);
fclose(outFile);
return 0;
}
```
示例:将二进制数据转换为ASCII文本文件(适用于小端序整数)
```c
include
include
int main() {
FILE *inFile = fopen("data.bin", "rb");
FILE *outFile = fopen("data_ascii.txt", "w");
if (inFile == NULL || outFile == NULL) {
perror("Error opening file");
return 1;
}
uint32_t *integers;
size_t numInts = fread(integers, sizeof(uint32_t), 10, inFile);
if (numInts != 10) {
perror("Error reading file");
fclose(inFile);
return 1;
}
for (size_t i = 0; i < numInts; ++i) {
fprintf(outFile, "%un", integers[i]);
}
fclose(inFile);
fclose(outFile);
return 0;
}
```
三、注意事项
使用 `fseek()` 可以定位文件指针,实现随机读写。
结构体需使用 `pragma pack` 或 `struct alignas` 确保按预期存储。
始终检查 `fopen`、`fwrite`、`fread` 等函数的返回值。
通过以上方法,可以灵活实现二进制数据的存储与转换,满足不同场景需求。