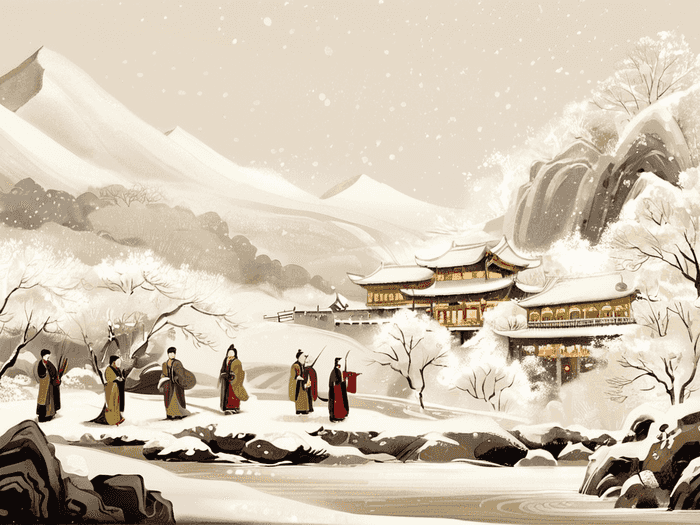
肖老师为您分享以下优质知识
将文件转换为二进制数据可以通过多种方式实现,具体方法取决于编程语言和需求。以下是常见的实现方式及示例代码:
一、使用编程语言内置函数
- 使用`FileInputStream`读取文件内容到字节数组,或使用`FileChannel`与`ByteBuffer`进行高效读写。
```java
public static byte[] fileToBytes(String path) throws IOException {
File file = new File(path);
FileInputStream fis = new FileInputStream(file);
FileChannel channel = fis.getChannel();
ByteBuffer buffer = ByteBuffer.allocate((int) channel.size());
channel.read(buffer);
buffer.flip();
byte[] data = new byte[buffer.remaining()];
buffer.get(data);
channel.close();
fis.close();
return data;
}
public static void bytesToFile(byte[] data, String path) throws IOException {
File file = new File(path);
FileOutputStream fos = new FileOutputStream(file);
ByteBuffer buffer = ByteBuffer.wrap(data);
buffer.flip();
fos.write(buffer.array());
fos.close();
}
```
Python
- 使用`open`函数以二进制模式读取文件,内容以`bytes`类型返回。
```python
def file_to_binary(path):
with open(path, 'rb') as f:
return f.read()
def binary_to_file(data, path):
with open(path, 'wb') as f:
f.write(data)
```
C++
- 使用`fstream`库,以`ios::binary`标志打开文件进行二进制读写。
```cpp
include
include
void binaryToFile(const std::string& data, const std::string& path) {
std::ofstream outFile(path, std::ios::binary);
outFile.write(data.c_str(), data.size());
}
std::string fileToBinary(const std::string& path) {
std::ifstream inFile(path, std::ios::binary);
std::string data((std::istreambuf_iterator(inFile)), std::istreambuf_iterator());
inFile.close();
return data;
}
```
二、使用系统命令
Linux/Mac
- 使用`xxd`或`od`命令将文件转换为十六进制或二进制表示。
```bash
转换为十六进制
xxd -p input.txt >
input.hex
转换为二进制(原始格式)
od -t x1
```
Windows
- 使用`dumpbin`工具查看二进制文件信息。
三、注意事项
大文件处理:
对于大文件,建议分块读取以避免内存溢出。例如Python中可以使用`open`的`read`方法指定块大小。
字符编码:文本文件需先转换为字节数组(如使用`encode`方法),再保存为二进制文件。
数据存储:二进制数据直接存储为0和1的序列,无法直接显示为可读文本。
通过以上方法,可根据具体需求选择合适的实现方式。若需进一步处理二进制数据(如数据库存储或网络传输),建议结合文件读写函数与二进制协议设计。