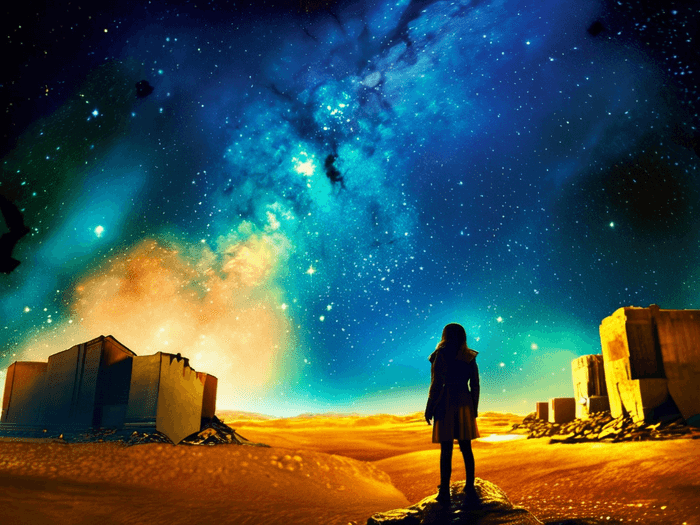
公务员全知道为您分享以下优质知识
将二进制数据转换为ASCII码在C语言中可以通过以下几种方法实现,具体选择取决于输入数据的格式和需求:
一、二进制文件转ASCII字符文件
读取二进制文件的每个字节,使用强制类型转换将其转换为对应的ASCII字符,然后写入新文件。例如:
```c
include
int main() {
FILE *input = fopen("binary.txt", "rb");
FILE *output = fopen("ascii.txt", "wb");
unsigned char byte;
if (input == NULL || output == NULL) {
perror("文件打开失败");
return 1;
}
while ((byte = fread(&byte, 1, 1, input)) != 0) {
putchar(byte);
}
fclose(input);
fclose(output);
return 0;
}
```
该方法直接将二进制数据(0-255)转换为ASCII字符输出。
按字(8位)处理
若二进制数据按字(8位)存储,可按每8位一组转换为ASCII字符。例如:
```c
include
int main() {
FILE *input = fopen("binary.txt", "rb");
FILE *output = fopen("ascii.txt", "wb");
unsigned char byte;
while (fread(&byte, 1, 1, input)) {
putchar(byte);
}
fclose(input);
fclose(output);
return 0;
}
```
该方法假设输入数据是8位对齐的。
二、二进制数据流转换为字符串(适用于网络数据包等场景)
若二进制数据是连续的位流(如网络数据包),需按每8位一组提取并转换为字符:
```c
include
include
int main() {
unsigned char *binary_data = (unsigned char *)malloc(1024); // 示例分配1KB内存
char *ascii_string = (char *)malloc(1024); // 预留足够空间存储转换后的字符串
int index = 0;
// 假设binary_data已填充二进制数据
for (int i = 0; i < 1024 / 8; i++) {
unsigned char byte = binary_data[i * 8];
ascii_string[index++] = (char)(byte & 0xFF);
if (index % 10 == 0) {
ascii_string[index] = '0'; // 每10个字符换行
}
}
ascii_string[index] = '0'; // 添加字符串结束符
printf("%sn", ascii_string);
free(binary_data);
free(ascii_string);
return 0;
}
```
三、其他注意事项
数据对齐:
确保二进制数据按8位对齐,否则可能导致未定义行为。可以使用位操作提取8位数据,例如:
```c
unsigned char byte = (binary_data[i * 8]