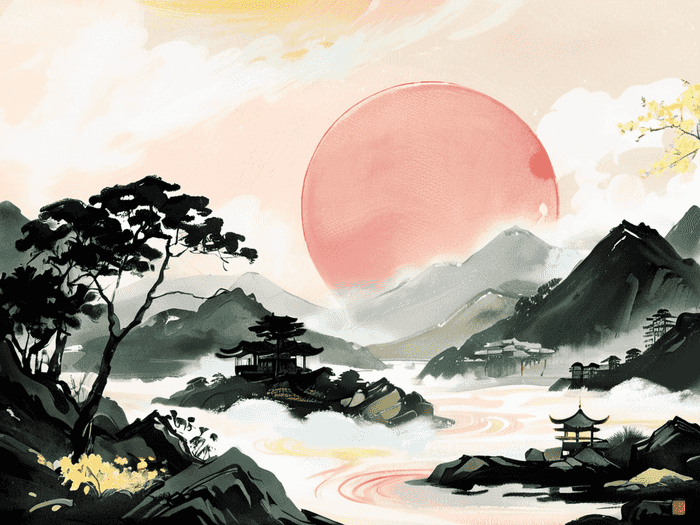
特立独行的兔子为您分享以下优质知识
在C语言中,通过二进制模式写入文件主要使用`fwrite()`函数。以下是具体方法和示例:
一、基本函数原型
```c
size_t fwrite(const void *ptr, size_t size, size_t nmemb, FILE *stream);
```
参数说明
`ptr`:指向要写入数据的指针
`size`:每个数据项的大小(以字节为单位)
`nmemb`:要写入的数据项数量
`stream`:指向目标文件的文件指针(如`FILE *fp`)
二、写入整型数组示例
```c
include
int main() {
int data[] = {1, 2, 3, 4, 5};
FILE *fp = fopen("output.bin", "wb");
if (fp == NULL) {
printf("Error opening filen");
return 1;
}
fwrite(data, sizeof(int), 5, fp);
fclose(fp);
return 0;
}
```
说明:将整型数组`data`以二进制形式写入`output.bin`文件,共写入5个整数。
三、写入结构体示例
```c
include
struct Student {
char name;
int age;
float height;
};
int main() {
struct Student student = {"Tom", 20, 1.75};
FILE *fp = fopen("student.bin", "wb");
if (fp == NULL) {
printf("Error opening filen");
return 1;
}
fwrite(&student, sizeof(struct Student), 1, fp);
fclose(fp);
return 0;
}
```
说明:将结构体`Student`以二进制形式写入`student.bin`文件,包含姓名、年龄和身高信息。
四、注意事项
- 使用`"wb"`模式以二进制写入文件,确保数据按原始格式存储,避免文本转换错误。
- 读取时需使用对应的`"rb"`模式。
数据对齐与字节序
- C语言默认使用 小端字节序(低位字节存储在低地址),而网络协议等场景可能使用 大端字节序。若需确保特定字节序,需手动处理(如使用`htonl`/`ntohl`转换整数)。
效率优化
- 对于大文件,建议使用缓冲区(如`unsigned char buf[MAXLEN]`)分块读写,避免一次性加载整个文件到内存。
五、完整示例:文件拷贝程序
```c
include
include
define MAXLEN 1024
int main(int argc, char *argv[]) {
if (argc < 3) {
printf("Usage: %s n", argv);
return 1;
}
FILE *infile = fopen(argv, "rb");
FILE *outfile = fopen(argv, "wb");
if (infile == NULL || outfile == NULL) {
printf("Error opening filesn");
return 1;
}
unsigned char buf[MAXLEN];
size_t bytes_read;
while ((bytes_read = fread(buf, sizeof(unsigned char), MAXLEN, infile)) >
0) {
fwrite(buf, sizeof(unsigned char), bytes_read, outfile);
}
if (bytes_read != MAXLEN) {
printf("Error reading filen");
return 1;
}
fclose(infile);
fclose(outfile);
return 0;
}
```
说明:
实现文件内容的二进制拷贝,支持大文件处理。
通过以上方法,可灵活实现C语言中二进制文件的写入操作,适用于存储结构化数据或非文本文件。