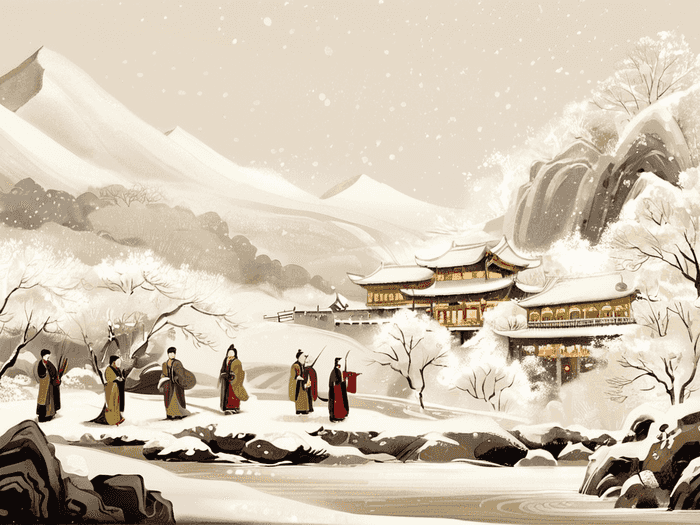
朱老师为您分享以下优质知识
在Python中读取二进制文件需要使用`open()`函数,并指定模式为`'rb'`(只读二进制模式)。以下是具体步骤和示例代码:
一、基础读取方法
使用`open()`函数以二进制模式打开文件,例如:
```python
with open('example.bin', 'rb') as file:
读取操作
pass
```
使用`with`语句可确保文件在操作完成后自动关闭,避免资源泄漏。
读取全部内容
使用`read()`方法可读取文件的全部内容,返回值为`bytes`类型:
```python
with open('example.bin', 'rb') as file:
binary_content = file.read()
print(binary_content)
```
若文件较大,建议使用分块读取以节省内存。
二、分块读取(推荐用于大文件)
当文件较大时,一次性读取整个文件可能占用过多内存。可通过指定`chunk_size`参数分块读取:
```python
chunk_size = 1024 每次读取1024字节
with open('example.bin', 'rb') as file:
while True:
data = file.read(chunk_size)
if not data:
break
处理数据,例如打印或写入其他文件
print(data)
```
三、读取特定部分
若需读取文件的前N个字节,可传递参数给`read()`方法:
```python
with open('example.bin', 'rb') as file:
first_10_bytes = file.read(10)
print(first_10_bytes)
```
四、示例综合应用
以下是一个完整的示例,展示如何读取二进制文件并解析其中的数据:
```python
import struct
def read_binary_file(file_path, num_bytes=10):
with open(file_path, 'rb') as file:
data = file.read(num_bytes)
使用 struct 模块解析二进制数据
unpacked_data = struct.unpack('h', data) 假设数据为2个短整型
return unpacked_data
示例调用
data = read_binary_file('example.bin')
print(data) 输出: (1, 2)
```
在解析二进制数据时,需根据数据格式选择合适的`struct`格式字符串(如`'h'`表示短整型)。
注意事项
数据类型转换:
二进制文件读取结果为`bytes`类型,若需使用其他数据类型,需进行转换(如使用`int()`、`float()`等)。
确保文件路径正确,若文件不存在会引发`FileNotFoundError`。
建议添加异常处理机制,例如:
```python
try:
with open('example.bin', 'rb') as file:
data = file.read()
except FileNotFoundError:
print("文件未找到")
except IOError as e:
print(f"读取文件时出错: {e}")
```
通过以上方法,可灵活地读取二进制文件内容,并根据需求进行后续处理。