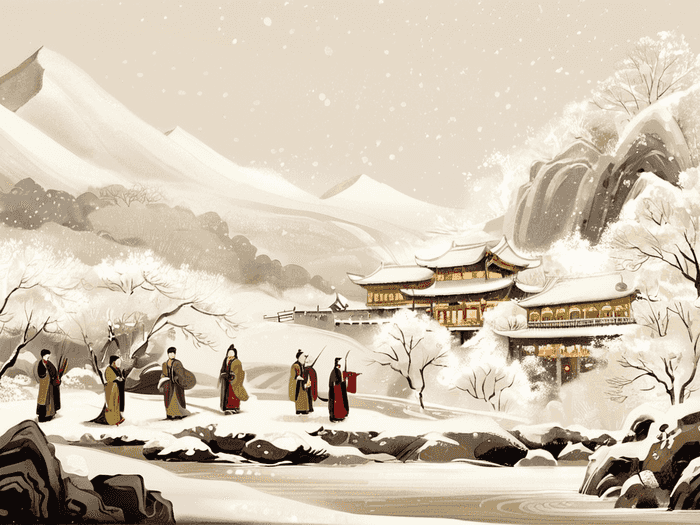
公考小黄为您分享以下优质知识
在C语言中读取二进制文件主要使用标准库函数`fopen`、`fread`和`fclose`。以下是具体步骤和示例代码:
一、核心步骤
使用`fopen`函数以二进制读取模式("rb")打开文件,返回文件指针。若失败则返回`NULL`。
读取数据
使用`fread`函数将文件内容读取到缓冲区或数组中。需指定数据类型、元素个数及文件指针。
关闭文件
使用`fclose`函数释放文件资源。
二、示例代码
以下是读取二进制文件到字符数组和整型数组的示例:
读取字符数组
```c
include
int main() {
FILE *file = fopen("example.bin", "rb");
if (file == NULL) {
printf("Error opening filen");
return 1;
}
char buffer;
size_t bytesRead = fread(buffer, sizeof(char), 100, file);
if (bytesRead == 0) {
printf("Failed to read filen");
fclose(file);
return 1;
}
buffer[bytesRead] = '0'; // 确保字符串终止
printf("File content: %sn", buffer);
fclose(file);
return 0;
}
```
读取整型数组
```c
include
int main() {
FILE *file = fopen("binary_file.bin", "rb");
if (file == NULL) {
printf("Error opening filen");
return 1;
}
int array;
size_t numElements = fread(array, sizeof(int), 100, file);
if (numElements == 0) {
printf("Failed to read filen");
fclose(file);
return 1;
}
for (int i = 0; i < numElements; i++) {
printf("%d ", array[i]);
}
printf("n");
fclose(file);
return 0;
}
```
三、注意事项
数据对齐:
二进制文件按固定格式存储,读取时需确保缓冲区大小与数据类型匹配。
错误处理:检查`fopen`和`fread`的返回值,确保操作成功。
文件关闭:操作完成后及时关闭文件,避免资源泄漏。
通过以上方法,可高效、安全地读取二进制文件内容。