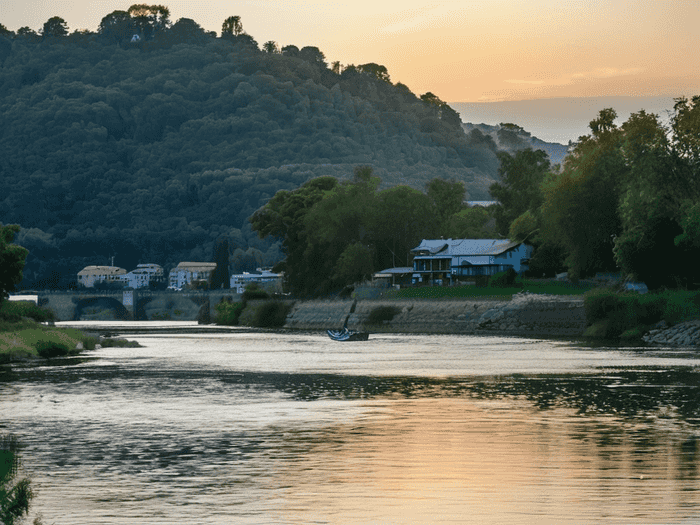
灯塔何老师为您分享以下优质知识
在C语言中输出二进制数据可以通过多种方法实现,以下是常见的几种方式:
一、使用 `printf` 函数
C语言提供了 `%b` 格式说明符,可以直接输出二进制形式(0和1)。
```c
include
int main() {
int num = 10;
printf("The binary representation of %d is %bn", num, num);
return 0;
}
```
输出:`The binary representation of 10 is 1010`
手动实现二进制输出
通过位运算逐位提取数字的二进制位,并格式化输出。
```c
include
void printBinary(int num) {
for (int i = sizeof(int) * 8 - 1; i >
= 0; i--) {
int bit = (num >
>
i) & 1;
printf("%d", bit);
if ((i + 1) % 8 == 0) printf(" ");
}
printf("n");
}
int main() {
int num = 10;
printf("Binary form: ");
printBinary(num);
return 0;
}
```
输出:`Binary form: 00000000000000000000000000001010`
二、使用库函数
`itoa` 函数
通过 `itoa` 函数将整数转换为二进制字符串(需包含 `` 头文件)。
```c
include
include
void printBinUsingItoa(int num) {
char binary;
itoa(num, binary, 2);
printf("Binary string: %sn", binary);
}
int main() {
int num = 10;
printBinUsingItoa(num);
return 0;
}
```
注意:`itoa` 在某些编译器中可能不支持,建议使用其他方法。
三、输出二进制文件
使用 `fwrite` 函数将二进制数据写入文件。
```c
include
int main() {
int data[] = {1, 2, 3, 4, 5};
FILE *fp = fopen("output.bin", "wb");
if (fp == NULL) {
printf("Error opening filen");
return 1;
}
fwrite(data, sizeof(int), 5, fp);
fclose(fp);
return 0;
}
```
运行后生成 `output.bin` 文件,内容为二进制数据。
四、其他方法
位域(Bit Field)
使用结构体中的位域定义特定二进制位。
```c
include
struct BitField {
unsigned int field1 : 4;
unsigned int field2 : 4;
};
int main() {
struct BitField bf = {5, 3};
printf("Field1: %04b Field2: %04bn", bf.field1, bf.field2);
return 0;
}
```
输出:`Field1: 101 Field2: 0011`
位运算符
通过位移和按位与操作直接构建二进制值。
总结
格式化输出:
优先使用 `%b` 或位运算实现,效率更高且代码简洁
字符串转换:需注意 `itoa` 的兼容性问题,推荐手动实现或使用其他库函数
文件操作:使用 `fwrite` 可批量写入二进制数据
根据具体需求选择合适的方法,例如调试时使用 `printf`,性能要求高时使用位操作或文件写入函数。