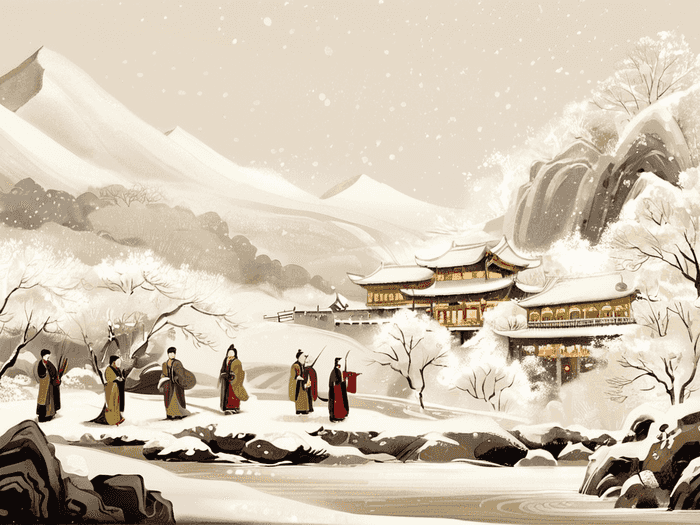
李老师求职指导为您分享以下优质知识
将文件转换为二进制格式通常涉及以下两种场景: 读取文件为二进制数据和 将二进制数据保存为文件。以下是针对不同编程语言的实现方法:
一、读取文件为二进制数据
Java实现
使用`FileInputStream`或`FileChannel`读取文件内容到字节数组中。
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
public class FileToBinaryConverter {
public static void main(String[] args) {
String filePath = "path/to/file.jpg";
try (FileInputStream fis = new FileInputStream(filePath);
FileChannel channel = fis.getChannel()) {
ByteBuffer buffer = ByteBuffer.allocate((int) channel.size());
channel.read(buffer);
byte[] binaryData = buffer.array();
// 处理binaryData
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
C语言实现
使用`ifstream`以二进制模式读取文件内容。
```c
include
include
int main() {
const char *filePath = "path/to/file.jpg";
FILE *file = fopen(filePath, "rb");
if (!file) {
perror("无法打开文件");
return 1;
}
fseek(file, 0, SEEK_END);
long fileSize = ftell(file);
fseek(file, 0, SEEK_SET);
char *buffer = malloc(fileSize);
if (!buffer) {
perror("内存分配失败");
fclose(file);
return 1;
}
fread(buffer, 1, fileSize, file);
fclose(file);
// 处理buffer
free(buffer);
return 0;
}
```
二、将二进制数据保存为文件
Java实现
使用`FileOutputStream`或`BinaryWriter`将字节数组写入文件。
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
public class BinaryToFileConverter {
public static void main(String[] args) {
String inputPath = "input.bin";
String outputPath = "output.bin";
byte[] data = {0x01, 0x02, 0x03}; // 示例数据
try (FileOutputStream fos = new FileOutputStream(outputPath);
ByteBuffer buffer = ByteBuffer.wrap(data)) {
buffer.flip(); // 切换为读模式
fos.write(buffer.array());
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
Python实现
使用`open`函数以二进制模式写入数据。
```python
def save_binary_file(file_path, data):
with open(file_path, 'wb') as f:
f.write(data)
示例数据
data = b'x01x02x03'
save_binary_file('output.bin', data)
```
三、注意事项
字符编码问题
- 文本文件在读取时需注意字符编码(如UTF-8、ASCII等),直接使用`FileReader`会按字符编码转换,而非二进制。若需获取原始二进制数据,建议使用`FileInputStream`或`FileChannel`。
- 若需将文本转换为二进制字符串,可使用编程语言提供的编码函数(如Java的`String.getBytes()`)。
二进制文件操作建议
- 大文件建议使用缓冲区(如`ByteBuffer`)分块读取,避免一次性占用过多内存。
- 数据库存储时,可将文件名、扩展名等元数据与二进制数据一起存储,便于后续检索。
以上方法覆盖了常见的文件二进制读写需求,可根据具体场景选择合适的语言和工具。